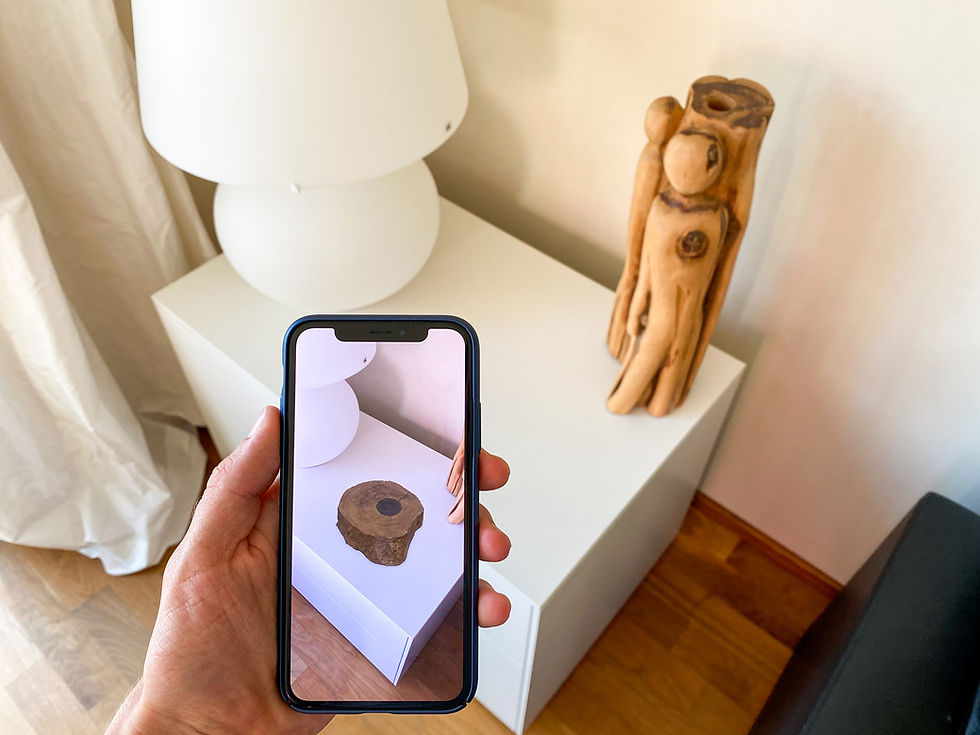
Augmented Reality (AR) has become an exciting technology that allows developers to create immersive experiences by overlaying virtual objects onto the real world. ARKit, Apple's framework for building AR applications, provides powerful tools and features to integrate AR into iOS apps using the Swift programming language.
In this blog post, we will explore how to use ARKit with Swift to create an AR application step by step.
Prerequisites
Before we dive into coding, make sure you have the following prerequisites:
A Mac running macOS 10.13.2 or later.
Xcode 9.0 or later.
An iOS device with an A9 or later processor, running iOS 11.0 or later.
Basic knowledge of Swift programming language and iOS app development.
Setting Up ARKit
To get started, let's create a new iOS project in Xcode and configure it for ARKit. Follow these steps:
Open Xcode and click on "Create a new Xcode project."
Choose "Augmented Reality App" template under the "App" category.
Enter the product name, organization identifier, and select Swift as the language.
Choose a location to save your project and click "Create."
Exploring the Project Structure
Once the project is created, let's take a quick look at the project structure:
AppDelegate.swift: The entry point of the application.
ViewController.swift: The default view controller for the ARKit app.
Main.storyboard: The user interface layout for the app.
Assets.xcassets: The asset catalog where you can add images and other resources.
Info.plist: The property list file that contains the configuration settings for the app.
Understanding the View Controller
The ViewController.swift file is the main view controller for our ARKit app. Open the file and let's explore its structure:
import UIKit
import ARKit
class ViewController: UIViewController {
@IBOutlet var sceneView: ARSCNView!
override func viewDidLoad() {
super.viewDidLoad()
// Set the view's delegate
sceneView.delegate = self
// Create a new scene
let scene = SCNScene()
// Set the scene to the view
sceneView.scene = scene
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
// Create a session configuration
let configuration = ARWorldTrackingConfiguration()
// Run the view's session
sceneView.session.run(configuration)
}
override func viewWillDisappear(_ animated: Bool) {
super.viewWillDisappear(animated)
// Pause the view's session
sceneView.session.pause()
}
}
extension ViewController: ARSCNViewDelegate {
}
The ViewController class inherits from UIViewController and conforms to the ARSCNViewDelegate protocol. It contains an ARSCNView object named sceneView, which is responsible for rendering the AR scene.
In the viewDidLoad() method, we set the sceneView delegate to self and create a new SCNScene object. We then assign the created scene to the sceneView.scene property.
In the viewWillAppear() method, we create an ARWorldTrackingConfiguration object, which is the primary configuration for AR experiences. We run the AR session by calling sceneView.session.run() with the created configuration.
Finally, in the viewWillDisappear() method, we pause the AR session by calling sceneView.session.pause().
Adding 3D Objects to the Scene
To add 3D objects to the AR scene, we need to implement the ARSCNViewDelegate methods. Modify the extension block in ViewController.swift as follows:
extension ViewController: ARSCNViewDelegate {
func renderer(_ renderer: SCNSceneRenderer, didAdd node: SCNNode, for anchor: ARAnchor) {
// Check if the added anchor is an ARPlaneAnchor
guard let planeAnchor = anchor as? ARPlaneAnchor else { return }
// Create a new plane node with the anchor's dimensions
let planeNode = createPlaneNode(with: planeAnchor)
// Add the plane node to the scene
node.addChildNode(planeNode)
}
private func createPlaneNode(with anchor: ARPlaneAnchor) -> SCNNode {
// Create a plane geometry with the anchor's dimensions
let planeGeometry = SCNPlane(width: CGFloat(anchor.extent.x), height: CGFloat(anchor.extent.z))
// Set the plane's color
planeGeometry.materials.first?.diffuse.contents = UIColor.blue.withAlphaComponent(0.5)
// Create a plane node with the geometry
let planeNode = SCNNode(geometry: planeGeometry)
// Position the plane node at the anchor's center
planeNode.position = SCNVector3(anchor.center.x, 0, anchor.center.z)
// Rotate the plane node to match the anchor's orientation
planeNode.eulerAngles.x = -.pi / 2
return planeNode
}
}
In the renderer(_:didAdd:for:) method, we check if the added anchor is an ARPlaneAnchor. If it is, we call the createPlaneNode(with:) method to create a plane node and add it to the scene.
The createPlaneNode(with:) method takes an ARPlaneAnchor as input and creates an SCNPlane geometry with the anchor's dimensions. We set the plane's color to blue with 50% transparency. Then, we create an SCNNode with the plane geometry, position it at the anchor's center, and rotate it to match the anchor's orientation. Finally, we return the plane node.
Running the AR App
Now that we have implemented the basic setup and added functionality to display plane nodes, let's run the AR app on a compatible iOS device. Follow these steps:
Connect your iOS device to your Mac.
Select your iOS device as the build destination in Xcode.
Click the "Play" button or press Command+R to build and run the app on your device.
Once the app is launched, point the camera at a flat surface, such as a tabletop or floor. As the ARKit detects and recognizes the surface, it will display a blue semi-transparent plane overlay on it.
Conclusion
In this blog post, we learned how to use ARKit with Swift to create an AR application in iOS. We explored the project structure, understood the view controller, and added 3D plane nodes to the scene using the ARSCNViewDelegate methods.
This is just the beginning of what you can achieve with ARKit. You can further enhance your AR app by adding custom 3D models, interactive gestures, and more.
Have fun exploring the possibilities of AR with Swift and ARKit!
Happy coding!
Comments