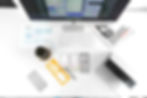
Testing is a critical aspect of software development, ensuring that your app works correctly from a user's perspective. In Android development, user interface (UI) testing is essential, and with the introduction of Jetpack Compose, a modern UI toolkit, testing UI components has become even more straightforward.
In this blog post, we will explore how to perform Espresso-based UI testing for Kotlin Android apps that utilize Jetpack Compose, providing code samples and best practices along the way.
Prerequisites
To follow along with this tutorial, you should have a basic understanding of Android app development using Kotlin, as well as familiarity with Jetpack Compose. Additionally, ensure that you have Android Studio installed on your machine.
Setting Up Espresso
1. Open your project in Android Studio.
2. In the build.gradle file of your app module, add the following dependencies:
androidTestImplementation 'androidx.test.espresso:espresso-core:<version>'
androidTestImplementation 'androidx.test.ext:junit:<version>'
Replace <version> with the appropriate version number of Espresso and JUnit.
3. Sync your project to download the required dependencies.
Writing Your First Espresso Test with Jetpack Compose
Let's create a simple Espresso test case to illustrate the basics of UI testing with Jetpack Compose. We'll write a test to verify the presence of a button and perform a click action on it.
1. Create a new Kotlin class called ExampleEspressoTest in your app's androidTest source set.
2. Import the necessary dependencies:
import androidx.compose.ui.test.*
import androidx.compose.ui.test.junit4.*
import androidx.test.espresso.Espresso.*
import androidx.test.espresso.matcher.ViewMatchers.*
import org.junit.Rule
import org.junit.Test
3. Define the test rule and launch the activity:
class ExampleEspressoTest {
@get:Rule
val composeTestRule = createComposeRule()
}
4. Write a simple test method:
@Test
fun testButtonVisibilityAndClick() {
// Launch the Compose screen/activity
composeTestRule.setContent {
// Compose UI code here
Button(
onClick = { /* Button click action */ }
) {
Text("Click Me")
}
}
// Check if the button is displayed
onView(withText("Click Me")).check(matches(isDisplayed()))
// Perform a click action on the button
onView(withText("Click Me")).perform(click())
}
5. Run the test using Android Studio's test runner or by executing the testButtonVisibilityAndClick method.
That's it! You've written your first Espresso test with Jetpack Compose. Let's explore more advanced features and techniques.
Working with Matchers and Actions
Espresso matchers and actions are still used in conjunction with Jetpack Compose. However, instead of targeting traditional Android views, we target Compose-specific components.
For example:
Matchers:
withText("text"): Matches a Compose component with the specified text.
isDisplayed(): Matches a Compose component that is currently visible on the screen.
Actions:
click(): Performs a click action on the Compose component.
Testing Jetpack Compose Components
When testing Compose components, you can use the onNode method to target specific components.
For example, to test a Button component:
onNode(hasText("Click Me")).performClick()
Verifying Assertions
Espresso assertions are still valuable for verifying conditions in Jetpack Compose. For example:
isDisplayed(): Checks if the Compose component is currently visible on the screen.
hasText("text"): Checks if the Compose component contains the specified text.
Conclusion
In this blog post, we explored how to perform Espresso-based UI testing for Kotlin Android apps using Jetpack Compose. We discussed setting up Espresso, writing your first test with Jetpack Compose components, and covered advanced topics like using matchers, actions, and verifying assertions.
Effective UI testing is crucial for ensuring the quality and reliability of your Android applications, especially when using Jetpack Compose. Espresso's powerful testing capabilities combined with Jetpack Compose's declarative UI approach provide a robust solution for UI testing in Kotlin-based Android projects.
Remember to continuously write and maintain UI tests as your app evolves to catch any regressions or issues.
Happy testing!