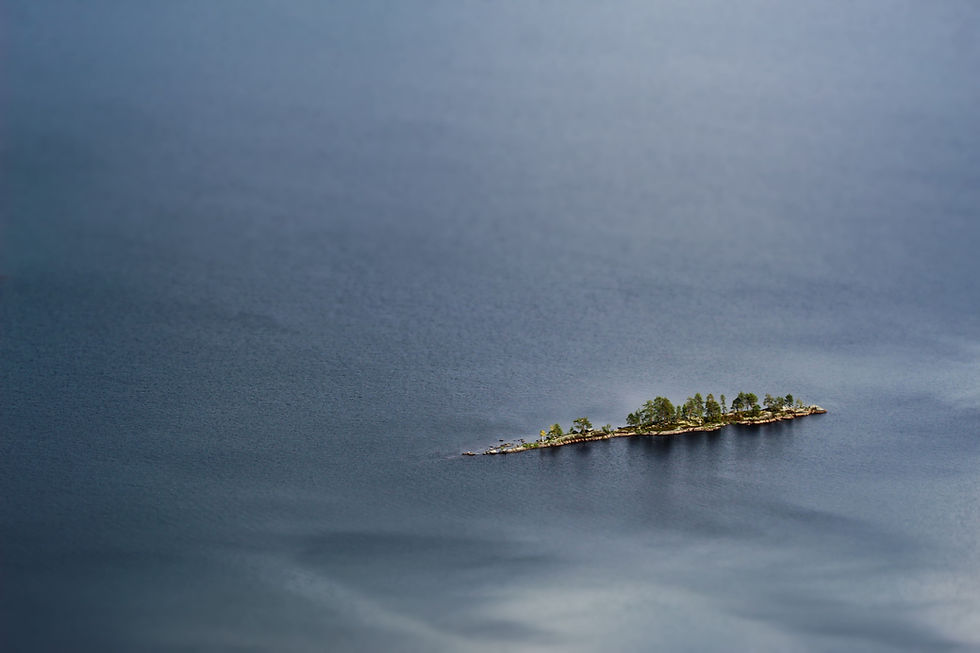
Isolates are a powerful feature of the Flutter framework that allow you to run code in separate threads. This can be useful for a variety of tasks, such as performing long-running operations or running code that is not safe to run on the main thread.
In this blog post, we will introduce you to isolates and show you how to use them in your Flutter apps. We will also discuss some of the best practices for using isolates.
What is an isolate?
An isolate is a thread that has its own memory space. This means that isolates can run code independently of each other and cannot share data directly.
Isolates are created using the Isolate class. The following code creates an isolate and starts a function in it:
import 'dart:isolate';
void main() {
// Create an isolate.
Isolate isolate = Isolate.spawn(_myFunction);
// Start the isolate.
isolate.resume();
}
void _myFunction() {
// This function will run in the isolate.
print('Hello from the isolate!');
}
The isolate is a separate thread of execution, so the code in the _myFunction() function will run independently of the code in the main thread.
The first line imports the dart:isolate library, which contains the classes and functions that are needed to create and manage isolates.
The main() function is the entry point for all Flutter applications. In this code snippet, the main() function creates an isolate and starts a function in it. The Isolate.spawn() function takes the name of the function to run in the isolate.
The _myFunction() function is the function that will be run in the isolate. The code in this function will run independently of the code in the main thread.
The isolate.resume() function starts the isolate. Once the isolate is started, the code in the _myFunction() function will start running.
When to use isolates
Isolates can be used for a variety of tasks, such as:
Performing long-running operations: Isolates are a great way to perform long-running operations that would otherwise block the main thread. For example, you could use an isolate to download a file from the internet or to process a large amount of data.
Running code that is not safe to run on the main thread: Some types of code are not safe to run on the main thread, such as code that accesses the file system or the network. In these cases, you can use an isolate to run the code in a separate thread.
Creating a multi-threaded application: Isolates can be used to create multi-threaded applications. This can be useful for applications that need to perform multiple tasks at the same time, such as a game or a video editor.
Best practices for using isolates
There are a few best practices to keep in mind when using isolates:
Avoid sharing data between isolates: As mentioned earlier, isolates cannot share data directly. If you need to share data between isolates, you can use a message passing system, such as the one provided by the dart:isolate library.
Use isolates sparingly: Isolates can add overhead to your application, so it is important to use them sparingly. Only use isolates when you need to perform a task that cannot be done on the main thread or when you need to create a multi-threaded application.
Test your code thoroughly: It is important to test your code thoroughly before using isolates in production. This is because isolates can be more difficult to debug than code that runs on the main thread.
Conclusion
Isolates are a powerful feature of the Flutter framework that allow you to run code in separate threads. This can be useful for a variety of tasks, such as performing long-running operations or running code that is not safe to run on the main thread.
If you have any questions, please feel free to leave a comment below.
Comments