Introduction to background modes in iOS apps
- Don Peter
- May 7, 2023
- 3 min read
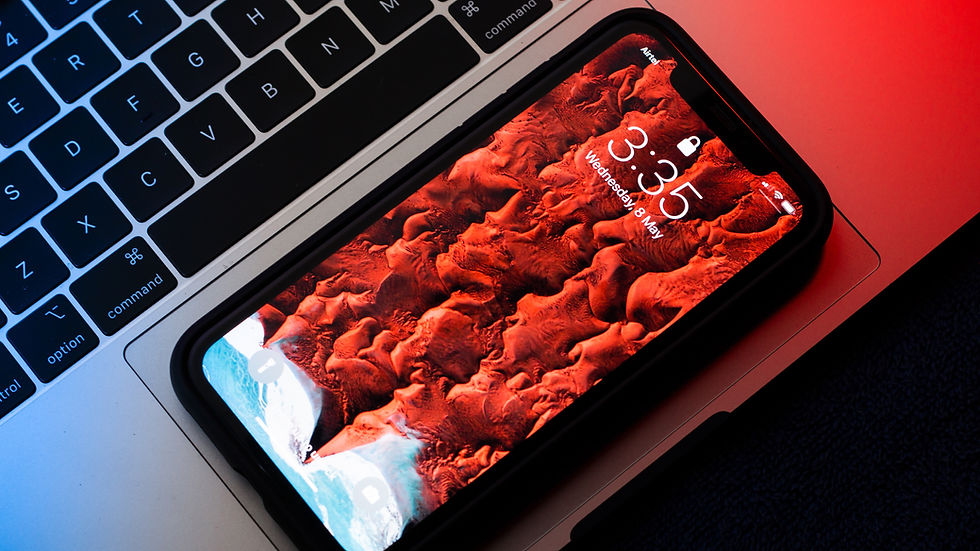
As an iOS developer, it's important to understand how to implement and use background modes in your app. Background modes allow your app to continue running in the background, even when the user has switched to another app or locked their device. This can be extremely useful for apps that need to perform tasks that take longer than the typical foreground time allowed by iOS.
In this blog post, we'll explore how to implement and use background modes in iOS apps.
Understanding Background Modes in iOS
Before we dive into how to implement background modes, it's important to understand what they are and what they can be used for. In iOS, background modes are a set of APIs that allow apps to continue running in the background for specific use cases. Some common examples of background modes include:
Audio: Allows your app to continue playing audio even when the app is in the background.
Location: Allows your app to receive location updates even when the app is in the background.
Background fetch: Allows your app to fetch new data in the background at regular intervals.
Implementing Background Modes
Implementing background modes in your iOS app requires a few steps. First, you'll need to enable the appropriate background mode in Xcode. To do this, go to the "Capabilities" tab for your app target and toggle on the appropriate background mode.
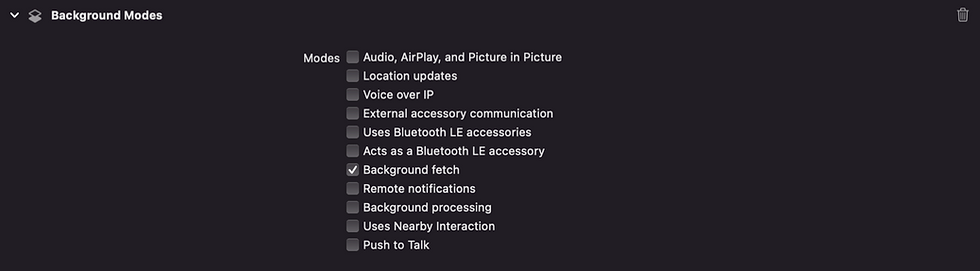
Next, you'll need to implement the appropriate code in your app to handle the background mode. For example, if you're implementing the "Audio" background mode, you'll need to make sure your app is configured to continue playing audio in the background. This may require some changes to your app's audio playback code.
import AVFoundation
class AudioPlayer {
let audioSession = AVAudioSession.sharedInstance()
var audioPlayer: AVAudioPlayer?
func playAudioInBackground() {
do {
try audioSession.setCategory(.playback, mode: .default, options: [.mixWithOthers, .allowAirPlay])
try audioSession.setActive(true)
UIApplication.shared.beginReceivingRemoteControlEvents()
let audioFilePath = Bundle.main.path(forResource: "audioFile", ofType: "mp3")
let audioFileUrl = URL(fileURLWithPath: audioFilePath!)
audioPlayer = try AVAudioPlayer(contentsOf: audioFileUrl, fileTypeHint: AVFileType.mp3.rawValue)
audioPlayer?.prepareToPlay()
audioPlayer?.play()
} catch {
print("Error playing audio in background: \(error.localizedDescription)")
}
}
}
In this code snippet, we create an AudioPlayer class that contains a function called playAudioInBackground(). This function sets the audio session category to .playback, which allows the app to continue playing audio in the background.
We also activate the audio session and begin receiving remote control events, which allows the user to control playback even when the app is in the background.
Finally, we load an audio file from the app's bundle and play it using an AVAudioPlayer instance. This allows the app to continue playing audio even when the app is in the background.
Note that this is just a simple example and there may be additional steps required depending on your specific use case. Be sure to consult Apple's documentation and guidelines for using the "Audio" background mode in your app.
Best Practices for Using Background Modes
While background modes can be extremely useful for certain types of apps, it's important to use them judiciously. Overuse of background modes can lead to increased battery drain and decreased device performance. Here are a few best practices for using background modes in your app:
Only enable the background modes that your app truly needs. Enabling unnecessary background modes can cause battery drain and decreased device performance.
Be mindful of how often your app uses background modes. If your app uses a lot of background modes, consider implementing a user-facing setting that allows the user to disable them if they choose.
Be sure to follow Apple's guidelines for using background modes. Apple has strict guidelines for using background modes in iOS apps, so be sure to familiarize yourself with these guidelines before implementing background modes in your app.
Testing Background Modes
Testing background modes in your iOS app can be challenging, since you'll need to test them while the app is running in the background. One way to test background modes is to use Xcode's "Simulate Background Fetch" feature. This allows you to simulate a background fetch event and test how your app responds.
Another way to test background modes is to run your app on a physical device and use the device for an extended period of time. This will allow you to test how your app behaves when running in the background for extended periods of time.
Conclusion
Implementing and using background modes in iOS apps can be extremely useful for certain use cases. However, it's important to use them judiciously and follow Apple's guidelines for using background modes. With the right approach, you can create iOS apps that continue to function even when the user is not actively using them.
Comments