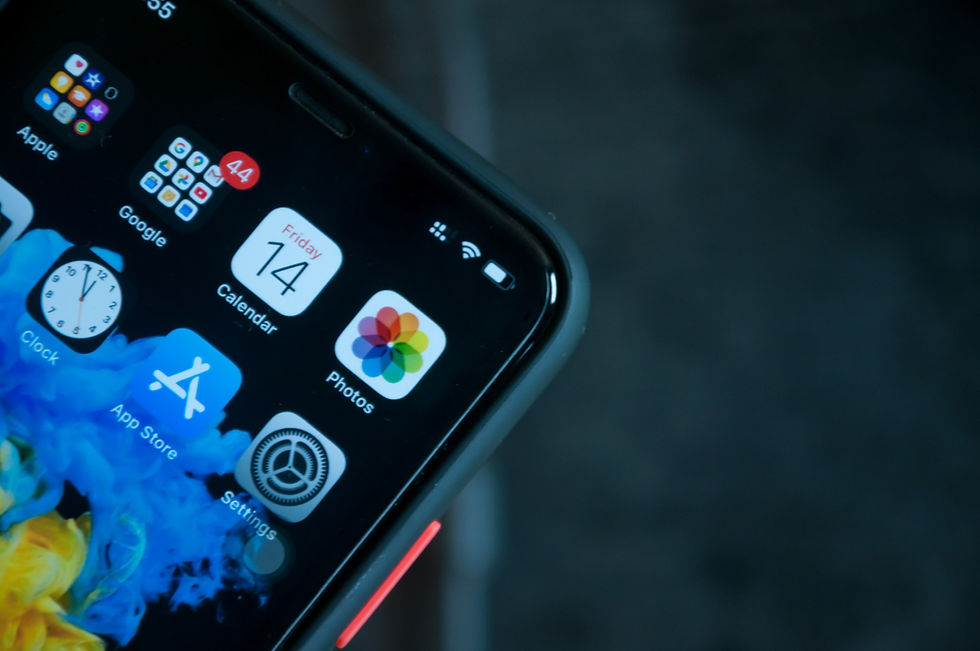
Creating a seamless user experience is an essential aspect of building a successful iOS app. Users expect apps to be fast, responsive, and intuitive.
In this blog post, we'll explore some Swift code examples that can help you create a seamless user experience in your iOS app.
Caching Data Locally in iOS App
One way to improve the performance of your app is to cache data locally. Caching data can reduce the need for repeated network requests, which can improve the speed of your app and create a smoother user experience.
In Swift, you can use the NSCache class to cache data in memory. NSCache is a collection that stores key-value pairs in memory and automatically removes objects when they are no longer needed.
Here's an example of how you can use NSCache to cache data in your app:
let cache = NSCache<NSString, NSData>()
func fetchData(from url: URL, completion: @escaping (Data?) -> Void) {
if let data = cache.object(forKey: url.absoluteString as NSString) {
completion(data as Data)
} else {
URLSession.shared.dataTask(with: url) { data, response, error in
if let data = data {
cache.setObject(data as NSData, forKey: url.absoluteString as NSString)
completion(data)
} else {
completion(nil)
}
}.resume()
}
}
In this example, we create an instance of NSCache and a function called fetchData that retrieves data from a URL. The function first checks if the data is already cached in memory using the cache's object(forKey:) method. If the data is found, the completion handler is called with the cached data. If the data is not found, we use URLSession to retrieve the data from the network. Once the data is retrieved, we cache it in memory using the cache's setObject(_:forKey:) method and call the completion handler with the data.
You can call this fetchData method whenever you need to retrieve data from the network. The first time the method is called for a particular URL, the data will be retrieved from the network and cached in memory. Subsequent calls to the method for the same URL will retrieve the data from the cache instead of the network, improving the performance of your app.
Handling Asynchronous Operations in Swift
Asynchronous operations, such as network requests and image loading, can sometimes cause a delay in your app's responsiveness. To prevent this, you can use asynchronous programming techniques to perform these operations without blocking the main thread.
1. Using closures
In Swift, one way to handle asynchronous operations is to use closures. Closures are blocks of code that can be passed around and executed at a later time. You can use closures to perform asynchronous operations and update the UI once the operation is complete.
Here's an example of how you can use closures to load an image asynchronously and update the UI once the image is loaded:
func loadImage(from url: URL, completion: @escaping (UIImage?) -> Void) {
URLSession.shared.dataTask(with: url) { data, response, error in
if let data = data {
let image = UIImage(data: data)
completion(image)
} else {
completion(nil)
}
}.resume()
}
In this example, we create a function called loadImage that loads an image from a URL. We use URLSession to retrieve the image data from the network. Once the data is retrieved, we create a UIImage object from the data and call the completion handler with the image. If there is an error retrieving the image data, we call the completion handler with nil.
You can call this loadImage method whenever you need to load an image asynchronously in your app. The completion handler allows you to update the UI with the loaded image once it's available.
2. Using DispatchQueue
Another way to handle asynchronous operations in Swift is by using the DispatchQueue class. DispatchQueue is a class that provides a way to perform work asynchronously on a background queue.
Here's an example of how you can use DispatchQueue to perform work on a background thread:
DispatchQueue.global().async {
// Perform background work hereDispatchQueue.main.async {
// Update the UI on the main thread
}
}
In this example, we use the global() method of DispatchQueue to get a reference to the global background queue. We call the async method to perform work asynchronously on the background queue. Once the work is complete, we use the main method of DispatchQueue to switch back to the main thread and update the UI.
You can use DispatchQueue to perform any work that doesn't need to be done on the main thread, such as data processing or database queries. By using a background thread, you can prevent the main thread from becoming blocked, which can improve the responsiveness of your app.
Using Animations in Swift
Animations can make your app feel more polished and responsive. In Swift, you can use the UIView.animate(withDuration:animations:) method to perform animations.
Here's an example of how you can use UIView.animate(withDuration:animations:) to fade in a view:
UIView.animate(withDuration: 0.5) {
view.alpha = 1.0
}
In this example, we use the animate(withDuration:animations:) method to animate the alpha property of a view. We specify a duration of 0.5 seconds for the animation. Inside the animation block, we set the alpha property of the view to 1.0, which will cause the view to fade in over 0.5 seconds.
You can use UIView.animate(withDuration:animations:) to animate any property of a view, such as its position or size. Animations can make your app feel more alive and responsive, which can improve the user experience.
Conclusion
Creating a seamless user experience is an essential aspect of building a successful iOS app. In this blog post, we explored some Swift code examples that can help you create a seamless user experience in your app.
We discussed caching data locally, handling asynchronous operations, and using animations. By using these techniques in your app, you can improve its performance, responsiveness, and polish, which can lead to happier users and a more successful app.
Comments