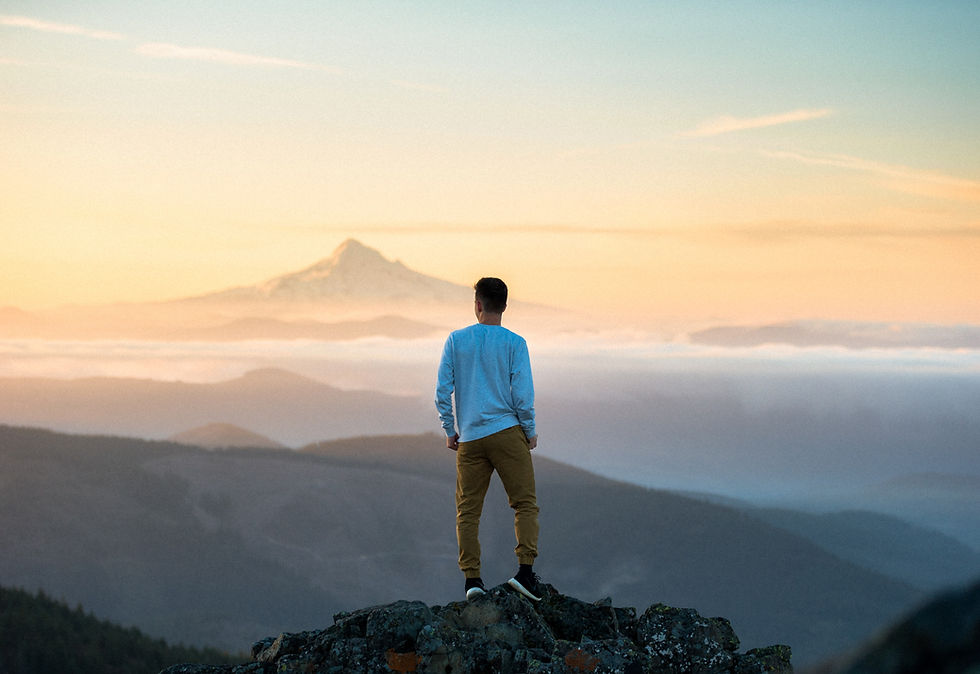
The General Data Protection Regulation (GDPR) is a European Union regulation that aims to protect the privacy and personal data of individuals. If your iOS app collects, processes, or stores personal data of EU residents, you must ensure that your app complies with GDPR.
In this guide, we will walk you through the steps to comply with GDPR in your iOS app, complete with Swift code samples.
1. Understand GDPR Principles
Before we dive into the technical aspects, it's crucial to understand the key principles of GDPR:
Consent: Users must give informed, explicit consent for their data to be collected and processed.
Data Minimization: Only collect and process data that is necessary for your app's functionality.
Data Portability: Users have the right to access and transfer their data.
Data Security: Implement robust security measures to protect user data.
Data Deletion: Allow users to delete their data upon request.
2. Inform Users with a Privacy Policy
Start by providing a clear and concise privacy policy within your app. You can display this during the onboarding process or in the app settings. Ensure that users can easily access and understand your privacy policy.
// Load and display the privacy policy HTML content
if let privacyPolicyURL = URL(string: "https://example.com/privacy-policy.html") {
let request = URLRequest(url: privacyPolicyURL)
webView.load(request)
}
3. Request Consent
To collect and process user data, you must obtain explicit consent. Create a consent dialog that explains why you need their data and how you will use it. Provide options for users to accept or reject data collection.
// Display a consent dialog
let alertController = UIAlertController(
title: "Data Collection Consent",
message: "We need your data to provide personalized recommendations. Do you consent?",
preferredStyle: .alert
)
let consentAction = UIAlertAction(title: "Consent", style: .default) { _ in
// User consented; start data collection
}
let rejectAction = UIAlertAction(title: "Reject", style: .destructive) { _ in
// User rejected data collection; handle accordingly
}
alertController.addAction(consentAction)
alertController.addAction(rejectAction)
present(alertController, animated: true, completion: nil)
4. Implement Data Minimization
Collect only the data necessary for your app's functionality. Avoid unnecessary data collection to minimize the risk of GDPR violations. Remove any unused data promptly.
5. Secure Data Storage
Protect user data by securely storing it. Use Apple's Keychain Services for sensitive data like passwords and tokens:
import Security
// Store a user's access token securely in the keychain
let accessToken = "user_access_token"
let accessTokenData = accessToken.data(using: .utf8)!
let keychainQuery = [
kSecClass as String: kSecClassGenericPassword as String,
kSecAttrAccount as String: "userAccessToken",
kSecValueData as String: accessTokenData
] as CFDictionary
let status = SecItemAdd(keychainQuery, nil)
if status == errSecSuccess {
print("Access token stored securely.")
}
6. Enable Data Portability
Implement a feature that allows users to access and export their data. Provide options to download data in common formats like JSON or CSV.
// Allow users to export their data
func exportUserData() {
// Prepare user data for export
let userData = ["name": "John Doe", "email": "johndoe@example.com"]
// Convert data to JSON
if let jsonData = try? JSONSerialization.data(withJSONObject: userData, options: []) {
// Offer the JSON file for download
let activityViewController = UIActivityViewController(activityItems: [jsonData], applicationActivities: nil)
present(activityViewController, animated: true, completion: nil)
}
}
7. Implement Data Deletion
Users have the right to request the deletion of their data. Create a feature to allow users to delete their accounts and associated data.
// Delete user account and data
func deleteUserAccount() {
// Perform data deletion// ...// Notify the user
let alertController = UIAlertController(
title: "Account Deleted",
message: "Your account and data have been deleted.",
preferredStyle: .alert
)
let okAction = UIAlertAction(title: "OK", style: .default) { _ in
// Handle user acknowledgment
}
alertController.addAction(okAction)
present(alertController, animated: true, completion: nil)
}
8. Handle Data Breaches
In the unfortunate event of a data breach, you must notify both users and authorities as required by GDPR. Implement a mechanism to detect and respond to breaches promptly.
9. Regularly Update and Audit
Stay compliant by regularly updating your app and privacy policy to reflect changes in data handling practices and GDPR regulations. Perform periodic data audits to ensure compliance.
Conclusion
Complying with GDPR in your iOS app is crucial to protect user privacy and avoid legal consequences. By following the principles of consent, data minimization, security, and providing user rights, you can build a GDPR-compliant app. Always stay informed about evolving GDPR regulations and adapt your app accordingly to maintain compliance.
Comments