Building iOS apps using SwiftUI
- Apr 6, 2023
- 3 min read
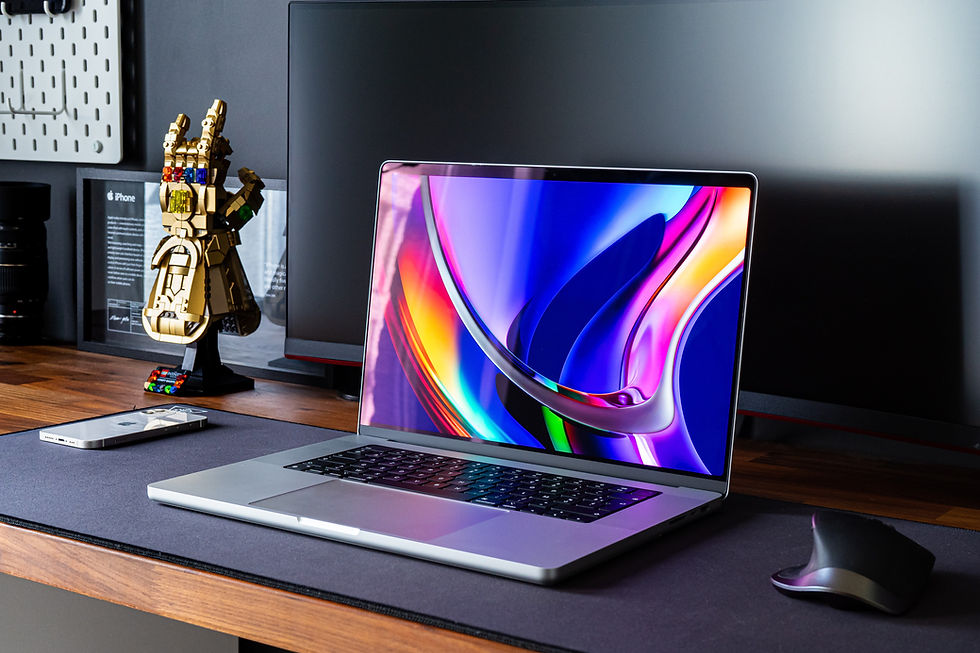
SwiftUI is a modern, declarative swift based framework for building user interfaces for iOS apps. It allows developers to create user interfaces using a simple, yet powerful syntax that is easy to read and write. In this article, we'll discuss how to build iOS apps using SwiftUI.
Step 1: Create a New SwiftUI Project
To create a new SwiftUI project,
Open Xcode and choose "File" > "New" > "Project".
Select "App" under "iOS", choose a template, and click "Next".
Give your project a name.
Select "SwiftUI" as the user interface, and click "Next".
Choose a location to save your project and click "Create".
Step 2: Understanding the Structure of a SwiftUI Project
When you create a new SwiftUI project, Xcode generates some boilerplate code for you.
The structure of a SwiftUI project consists of three main files:
ContentView.swift: This is the main view of your app. It's where you'll define the layout and behavior of your user interface.
App.swift: This file defines the entry point of your app.
SceneDelegate.swift: This file sets up the initial scene of your app and sets the root view controller to your main view.
Step 3: Building the User Interface
To build the user interface of your app, you'll use SwiftUI's declarative syntax. This means you'll declare what your user interface should look like, and SwiftUI will handle the rest. Let's create a simple user interface with a button and a text view.
In ContentView.swift, replace the existing code with the following:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("Welcome to my app!")
.font(.title)
.padding()
Button("Tap me!") {
print("Button tapped!")
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
In this example, we've defined a vertical stack (VStack) that contains a text view (Text) and a button (Button). The text view has a font size of .title and some padding. The button has a label of "Tap me!" and a closure that prints "Button tapped!" to the console when tapped.
Step 4: Running the App
To run the app, select "Product" > "Run" from the menu, or press Command-R. Xcode will build and run the app in the simulator. You should see the text "Welcome to my app!" and a button labeled "Tap me!". When you tap the button, "Button tapped!" should be printed to the console.
Step 5: Adding Navigation
SwiftUI makes it easy to add navigation to your app. Let's add a navigation view and a navigation link to our app.
Update ContentView.swift with the following:
struct ContentView: View {
var body: some View {
NavigationView {
VStack {
Text("Welcome to my app!")
.font(.title)
.padding()
NavigationLink(destination: Text("Second view")) {
Text("Go to second view")
}
}
.navigationBarTitle("My App")
}
}
}
In this example, we've wrapped our content in a NavigationView. We've also added a NavigationLink that takes the user to a second view when tapped. The second view is just a text view that says "Second view".
That's it! You've just built a simple iOS app using SwiftUI.
SwiftUI is a powerful and flexible framework that can help you build beautiful user interfaces for your iOS apps with ease. With SwiftUI, you can focus on the structure and layout of your UI, rather than the implementation details.
SwiftUI provides a lot of built-in controls and views that make it easy to build complex UIs. You can also create your own custom views and controls to further customize your app's user interface.
In this article, we've covered the basics of building an iOS app using SwiftUI. We've created a simple user interface with a button and a text view, added navigation to our app, and explored the structure of a SwiftUI project.
SwiftUI is a powerful and intuitive framework that simplifies the process of building user interfaces for iOS apps. It's a great tool for developers who want to create beautiful, responsive, and dynamic user interfaces quickly and efficiently. If you haven't already, give SwiftUI a try and see how it can help you create stunning iOS apps!
Comments