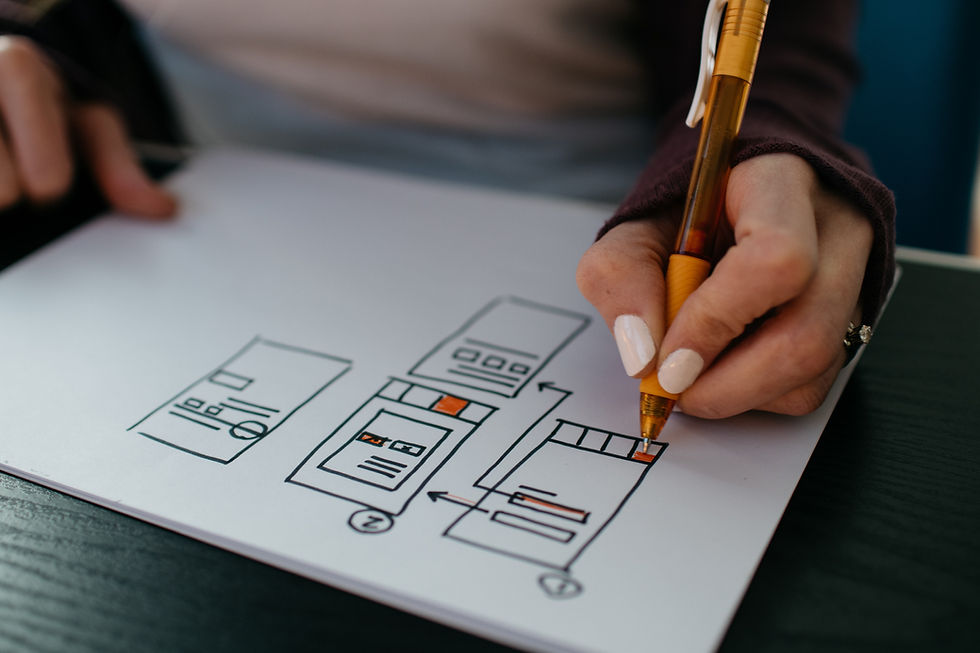
Flutter is a powerful framework that allows developers to create beautiful, responsive user interfaces (UI) for mobile and web applications. Flutter's LayoutBuilder widget is an essential tool for building responsive UIs, as it allows developers to customize their layouts based on the available space on a device's screen.
In this blog post, we'll explore how to use Flutter's LayoutBuilder widget to build responsive UIs that can adapt to different screen sizes and orientations.
What is the LayoutBuilder Widget?
The LayoutBuilder widget is a powerful tool in Flutter that allows you to customize the layout of your widgets based on the available space on a device's screen. It provides a way to build responsive UIs that can adapt to different screen sizes and orientations.
The LayoutBuilder widget works by providing you with a BoxConstraints object that describes the minimum and maximum constraints for the widget's size. You can use these constraints to build a UI that can adapt to different screen sizes.
How to Use the LayoutBuilder Widget
To use the LayoutBuilder widget, you'll need to wrap it around the widget that you want to make responsive. Let's say you want to create a responsive container that changes its size based on the available space on the screen. You can achieve this by using the LayoutBuilder widget like this:
LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return Container(
height: constraints.maxHeight * 0.5,
width: constraints.maxWidth * 0.5,
color: Colors.blue,
);
},
);
In this example, we've wrapped the Container widget with the LayoutBuilder widget. Inside the builder function, we use the BoxConstraints object to set the height and width of the container. We're multiplying the available height and width by 0.5 to ensure that the container is half the size of the available space.
Building a Responsive UI with LayoutBuilder
Now that we've seen how to use the LayoutBuilder widget, let's explore how to build a responsive UI using it.
Let's say we want to create a responsive layout that adapts to different screen sizes and orientations. We'll start by creating a simple UI with a text widget and an image widget.
class ResponsiveLayout extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Responsive Layout'),
),
body: Column(
children: [
Text(
'Welcome to our app',
style: TextStyle(fontSize: 24),
),
Image.network(
'https://via.placeholder.com/350x150',
),
],
),
);
}
}
This UI consists of a column with a text widget and an image widget. The text widget displays a welcome message, and the image widget displays an image.
Next, we'll wrap the column widget with the LayoutBuilder widget. Inside the builder function, we'll use the BoxConstraints object to determine the available space on the screen.
class ResponsiveLayout extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Responsive Layout'),
),
body: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return Column(
children: [
Text(
'Welcome to our app',
style: TextStyle(fontSize: constraints.maxWidth * 0.05),
),
Image.network(
'https://via.placeholder.com/350x150',
height: constraints.maxHeight * 0.5,
),
],
);
},
),
);
}
}
In the example above, we've wrapped the Column widget with the Layout Builder widget. Inside the builder function, we're using the BoxConstraints object to set the font size of the text widget based on the available width. We're also setting the height of the image widget based on the available height.
By doing this, our UI will adapt to different screen sizes and orientations. If the screen is larger, the text and image will be larger. If the screen is smaller, the text and image will be smaller.
Additional Tips for Building Responsive UIs with Flutter
Here are a few additional tips to help you build responsive UIs with Flutter:
Use MediaQuery: The MediaQuery widget provides information about the device's screen size and orientation. You can use it to set the font size, padding, and margins of your widgets based on the device's screen size.
Use Expanded and Flexible Widgets: The Expanded and Flexible widgets allow your widgets to expand or shrink based on the available space. You can use them to create layouts that adapt to different screen sizes.
Use OrientationBuilder: The OrientationBuilder widget provides information about the device's orientation. You can use it to change the layout of your UI based on the device's orientation.
Test on Different Devices: It's important to test your UI on different devices to ensure that it looks good on all screen sizes and orientations.
Conclusion
Flutter's LayoutBuilder widget is a powerful tool for building responsive UIs that can adapt to different screen sizes and orientations. By using the BoxConstraints object provided by the LayoutBuilder widget, you can customize the layout of your widgets based on the available space on the screen. By following the additional tips listed above, you can create beautiful, responsive UIs that look great on any device.
Comments